Unreal Engine 4.25 Preview
The other day, the Preview of UE4 4.25 became available for download.
(As of writing this article, it is Preview 3)
The contents are described on the following page.
Unreal Engine 4.25 Preview - Unreal Engine Forums
Among the many updates, the following item caught my eye. (The FProperty Refactor… part)

The content is “From 4.25, UProperty has become FProperty and is no longer a UObject.”
Until now, UProperty inherited from UObject. In UE4, classes inheriting from UObject have a naming convention starting with U. Hence the class name UProperty.
- Reference [Coding Standard](https://docs.unrealengine.com/en-US/Programming/Development/CodingStandard/index.html)
Since it no longer inherits from UObject (it now inherits from a class called FField), the class name changed to FProperty.
What is UProperty?
First of all, what is the UProperty (FProperty) that was changed this time? It is information about member variables or function arguments of a C++ class created by the UE4 property system.
Details are explained thoroughly on the official page below.
- Reference [Unreal Property System (Reflection)](https://www.unrealengine.com/en-US/blog/unreal-property-system-reflection)
Furthermore, UProperty is derived into UStringProperty, UBoolProperty, etc., depending on the property type.
It is used for various purposes within the UE4 engine, but from the perspective of us UE4 users, it is used when we want to do things like the following:
- Access properties of an object defined in Blueprint from the C++ side
- Create a custom serializer for an object
- Manipulate object properties by specifying the property name
Using the UE4 property system with UProperty like this allows for building mechanisms to manipulate objects more flexibly.
(Reference) Example of getting the values of all properties of an object
The following code gets the values of all properties of Obj.
(* This example assumes that the type of all properties is uint8)
for (TFieldIterator<UProperty> PropIt(Obj->GetClass()); PropIt; ++PropIt)
{
UProperty* Property = *PropIt;
// Get property name
FString PropertyName = Property->GetName();
// Get property value
uint8 CurrentPropAddr = *(PropIt->ContainerPtrToValuePtr<uint8>(Obj));
}
Why was it changed to FProperty?
I haven’t followed all the information, so this includes a lot of personal speculation, but I think a major reason was to remove it from the management scope of GC (Garbage Collection) by making it not a UObject.
When an instance is allocated in memory as a UObject, the GC checks whether the instance should be destroyed at the appropriate timing. Instances determined to be destroyable by the check result will be destroyed.
If the number of UObject instances in the entire project is large, the search time for the GC to check for destroyable targets increases. (Because the absolute number of items to check increases)
Increased search time can lead to severe adverse effects, such as the frame rate dropping sharply at the GC check timing (experienced this).
Therefore, although it depends on the case, having fewer UObject instances is generally considered better for performance.
Building conventional (up to 4.24) C++ code with 4.25
I tried building the code that enumerates object properties written earlier with 4.25.
It didn’t result in an error, but the following Warning appeared.
warning C4996: UProperty has been renamed to FProperty Please update your code to the new API before upgrading to the next release, otherwise your project will no longer compile.
The Warning says, “UProperty has changed to FProperty, so change it quickly.” However, being a Warning means it will still work for now.
The reason for this can be understood by looking at the definition of UProperty in 4.25.
It was defined as follows in the header DefineUPropertyMacros.h
.
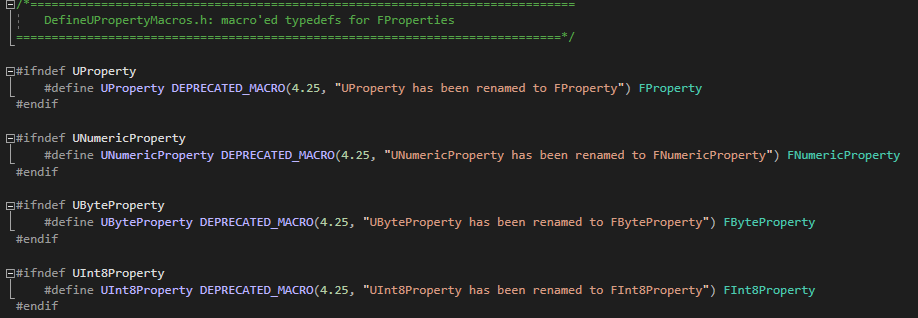
All UProperty are set to be replaced with FProperty.
Since DEPRECATED_MACRO is also embedded during this replacement, the previous Warning seems to appear.
So, does this mean that code using UProperty can be left as is for the time being? It seems not.
It’s just being replaced; the UProperty written in the C++ code is already not a UObject, so code like the following will result in an error.
UProperty* Property = *PropIt;
if (UBoolProperty* BoolProperty = Cast<UBoolProperty>(Property))
{
bool bValue = BoolProperty->GetPropertyValue(Obj);
}
The code above means “if the type of a certain property is bool, get the value as bool.”
In this, Cast<UBoolProperty>(Property)
is used to check if UProperty can be cast to UBoolProperty, but Cast can only be used for UObjects.
Therefore, building this code results in an error.
(* In the example above, the same can be achieved with FProperty by replacing it with the CastField function)
For the same reason, IsValid
and similar functions are likely to become unusable.
Additionally, if there are parts where UProperty is passed as a UObject argument to functions, they need to be changed appropriately.
Summary
- From 4.25, UProperty became FProperty
- Replace箇所 where U~Property is used in C++ with F~Property
- Check not only for replacement but also if UProperty is used as UObject anywhere
Bonus
Since the old UProperty definition remains in UnrealTypePrivate.h, it feels like UProperty could be extended for a while longer by including this header, but it seems better not to use it as it would deviate from the proper flow.